Top 30 Flutter Interview Questions and Answers 2024
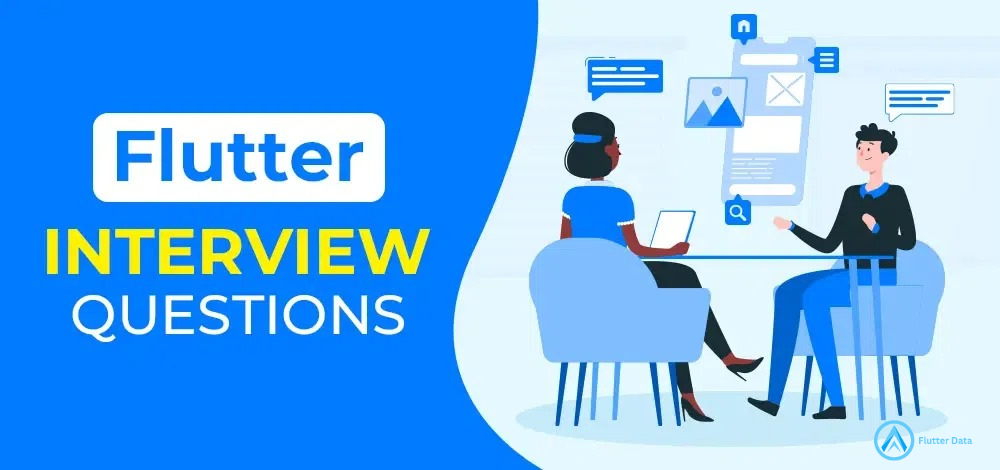
Flutter, the popular cross-platform mobile development framework, has taken the industry by storm. Its ability to build stunning and performant apps for both iOS and Android with a single codebase makes it a highly sought-after skill for developers.
If you’re aiming to land your dream Flutter developer role, nailing the interview is crucial. Flutter interview questions typically cover core concepts like widgets, state management, hot reload, and Dart programming. Understanding these fundamentals and practicing your answers will significantly increase your chances of success.
Flutter Interview Questions
1. What is Dart?
- In Flutter Dart is a general-purpose, object-oriented programming (oop) language featuring with C-style syntax. It is open- source and developed by Google in 2011. The Dart language can be compiled both AOT (Ahead- of-Time) and JIT (Just-in-Time.
2. What is Flutter?
- The most important question in flutter interview questions is that Flutter is a framework developed by Google that allows you to build natively compiled mobile applications with one programming language and a single codebase. Flutter is not a language; it is an SDK. Flutter framework apps use Dart programming language for creating the apps in Android , IOS, PWA and other platforms. Flutter is mainly optimized for 2D mobile apps that can run on both Android, iOS, PWA, window and other platforms.
3. What is the Architecture of Flutter?
- Flutter has a modular, layered architecture. This method allows you to write your application logic once, ensuring it behaves consistently across all platforms, even though the underlying engine code differs for each one. The layered architecture also offers various opportunities for customization and overrides where necessary.
4. What are the different Build modes in Flutter?
- This is most important question in flutter interview questions
- There are three main types of build modes in Flutter:
- Debug: This mode is used for testing and development purposes. It allows you to use debugging tools and view additional information about your app.
- Profile: This mode balances debugging capabilities with performance optimization. It’s useful for profiling your app’s performance and identifying potential bottlenecks.
- Release: This mode is used for deploying your app to the App Store or Google Play. It is optimized for performance and has debugging features disabled.
5. What types of Tests can you perform in Flutter?
- Unit Tests: It tests a single function, method, or class in Flutter code. The objective is to guarantee that the code functions correctly in diverse conditions. This testing is used for checking the validity of business logic.
- Widget Tests: It tests a single widget. Its goal is to ensure that the widget’s UI looks and interacts with other widgets as expected.
- Integration Tests: These tests validate a complete application or a significant portion of it. Their goal is to ensure that all widgets and services interact and function together as expected.
6. What is the difference between AOT & JIT in Flutter?
- In development mode, Flutter is compiled just-in-time. This is why we can perform hot-reload and hot-restart so quickly. Release mode, your code is compiled ahead-of-time, to native code. It is for better performance, minimizes size and removes other stuff that are useful only in dev mode.
7. How does Dart AOT work?
- Flutter Dart source code will be translated into assembly files, then assembly files will be compiled into binary code for different architectures by the assembler in the Flutter Data.
- For mobile applications, the source code is compiled for multiple processors—ARM, ARM64, and x64—and for both Android, iOS, PWA, window and other platforms. This results in multiple binary files for each supported processor and platform combination.
- Flutter Beginner Tutorial For Mobile App Developers
8. What’s the difference between Hot Reload and Hot Restart?
- This is most important question in flutter interview questions
- In Flutter, hot reload can update UI code almost instantly while maintaining application state in Flutter data. Hot restart, by comparison, takes some long time to run the app code because it resets the app state to its initial conditions before updating the UI code. Both are faster than doing a Full restart, which requires recompiling the app.
9. Why does a Flutter app usually takes longer to execute when running for the first time?
- The App run first time you build a Flutter application, it takes much longer than usual since Flutter data creates a device-specific IPA or APK file. XCode and Gradle build are used in this process to build a files, which usually takes a lot of time on first time run.
10.What is a Widget? and how many types of widgets are there in Flutter?
- A Flutter widget is a high-level object used to describe any part of a Flutter data application. It can be but is not limited to UI elements, layout (alignment, padding, …), data (theme, configurations, …). In flutter everything is a widget, almost everything you’ll code will be inside a Widget. There are 2 types of widgets in Flutter, Stateless & Stateful widgets.
11.What is the difference between Stateless & Stateful widgets in Flutter?
- StatelessWidget is an immutable class in Flutter that acts as a blueprint for some part of the UI layout in Flutter data. You use it when the widget doesn’t change any value while displaying and, therefore, has no State.
- StatefulWidget is also immutable, It has initstate and it’s coupled with a State Object that allows you to rebuilding with new values whenever setState() is called.
12.What is the difference between WidgetsApp and MaterialApp?
- WidgetsApp provides basic navigation. Alongside the widgets library, it encompasses many fundamental widgets essential to Flutter’s functionality.
- MaterialApp and its corresponding material library are constructed as a layer above WidgetsApp and the widgets library. They implement Material Design, providing the application with a consistent appearance and behavior across various platforms and devices.You can also use CupertinoApp to make iOS users feel at home, and you can also build your own set of custom widgets classes to fit your brand in flutter data.
13.Can you nest a Scaffold? Why or why not?
- Yes, you can nest a Scaffold. In flutter Scaffold is just a widget, so you can add it anywhere in Flutter a widget might go. By nesting a Scaffold In code, you can layer drawers in scaffold, also snack bars and bottom sheets in flutter.
14.What is async & await in Flutter?
- Await suspends the flow until the async method completes in Flutter Data. Await generally means: Wait here until this function is finished so that you can get its return value. It will be used with async with the function .
15. What is the difference between a Future and a Stream?
- This is most important question in flutter interview questions
- A Stream is a combination of Futures. Future builder has only one response when call, but Stream builder could have any number of Responses when it will be call.
16.What is a Stream?
- It is a sequence of asynchronous data. We can create a stream using async generator (async*) and listen to it using listen(). There are 2 types of streams, Single Subscription and Broadcast streams.
17.What are the different types of Streams?
- Single Subscription Streams: These streams deliver events sequentially. They are regarded as sequences that are part of a larger entity.These streams are used when the order in which events are received matters, such as reading a file. There can be only one listener in Flutter throughout the sequence, and without a listener function, the event won’t be call and get a data.
- Broadcast Streams: These streams distribute events to their subscribers. When subscribers subscribe to events, they can immediately start listening to them. Broadcast streams are versatile, enabling multiple listeners to listen simultaneously. Additionally, one can resume listening even after canceling a previous subscription.
18.What is BuildContext and how is it useful?
- It is actually the widget’s element in the Element tree, so every widget has its own BuildContext. You usually use it to get a reference to the theme or to another widget. Put simply, if you want to show a material dialog, you need to have access to the scaffold. In Flutter data You can get it with Scaffold.of(context) in Flutter code , where context is the build context. of() method reference up the widget tree until it locates the nearest scaffold in Flutter.
19.What is the difference between main() and runApp()?
- This is most important question in flutter interview questions
- main() is needed for every Dart program since it’s the entry point for the app. But in Flutter apps, you should also call runApp() to load the framework & attach the given widget to screen.
20.What is dispose() method in flutter?
- It is used to release the memory allocated to variables when state object is removed from the tree permanently. After the function calls dispose, the State manegment object is considered unmounted, and In Flutter the mounted property is set to false and in Flutter data It is an error to call setState() Function at this point. Once a State object has been disposed, it reaches a terminal stage in its lifecycle, meaning there’s no way to remount it.
21.What are the different types of Image widgets in flutter?
- Image(): for obtaining an image from an ImageProvider. Image.asset(): Used to obtain an image from an AssetBundle using a key.
- Image.network(): Used to fetch an image from a URL.
- Image.file(): Used to retrieve an image from a File.
- Image.memory(): Used to acquire an image from a Uint8List.
22.What is the difference between Required and Optional parameters?
- Required parameters are the arguments essential for the function to complete its code block.
- While Optional parameters doesn’t have to be specified by the caller while calling the function. Optional parameters can only be declared after required parameters. It can be Positional [ ] or Named { }, it can also have a default value, which is used when a caller does not specify a value.
23.What is MediaQuery?
- It provides a higher-level view of the current app’s screen size and can also give more detailed information about the device screen resolution and its layout preferences.
24.Why do we need Mixins?
- Dart does not support multiple inheritances. Thus, to implement multiple inheritances in Flutter/Dart, we need mixins. Mixins is provide a way to the write the reusable widgets code in multiple class hierarchies.
25.What are Keys in Flutter, and when to use it?
- Keys in Flutter are used as an identifier for Widgets, Elements and Semantics Nodes. When a new widget aims to update an existing element, we can utilize keys by ensuring the new widget’s key matches the current widget key associated with the element. It’s crucial that keys are consistent among elements within the same parent—they shouldn’t differ.
- Subclasses of Key must either be a Global Key or a Local Key. Keys are particularly handy when manipulating a collection of widgets of the same type, facilitating actions like adding, removing, or reordering, especially when those widgets hold state.
26.What is an event loop, and how is it related to isolates?
- Dart code runs on a single thread called an isolate. Separate isolates don’t share any memory and they only communicate through messages sent over ports.
- Every isolate function has an event loop, which schedules time for asynchronous tasks to run. The tasks can be on one of two different queues: the microtask queue or the event queue. Micro tasks always run first, but they are mainly internal tasks that the developer doesn’t need to worry about. Calling a future puts the task on the event queue when the future completes.
- A lot of new Dart programmers think async methods run on a separate thread. Although that may be true for I/O operations that the system handles, it isn’t the case for your own code. That’s why if you have an expensive computation, you need to run it on a separate isolate.
27.What is Tween animation?
- A shortened version of Flutter an in-between animation is a tween animation in Flutter animation. In tween animation, both the starting and ending points of an animation must be defined. With this approach, the animation starts from the beginning and transitions through a series of values until it reaches the endpoint. Transition speed and duration are also determined by using the tween animation.
28.What is Ticker and why we use in Flutter?
- We use code to show how often animations are updated in Flutter Data. This type of signal-sending class sends signals at a constant frequency, typically 60 times per second. It’s akin to the constant ticking of a watch. For each tick, a callback method is triggered, providing the time elapsed since the first tick and each second thereafter since its initiation. These tickers synchronize instantly, even if they start at different times.
29.What is meant by Null-aware operators?
- Null-aware operators allow you to make computations based on whether or not a value is null. Dart offers helpful tools for managing null values.
- The “??=” assignment operator: It assign a data to a variable only if the variable value is currently null .The “??” null-aware operator: Expression 1 is checked first for nullness, and if it is not null, its value is returned; otherwise, expression 2 is evaluated, and its value is returned.
- The “?.” The Safe Navigation Operator Sign: It is also known as the Elvis operator in the Flutter . It is possible to use the ?. operator when calling a method/getter on an object, as long as the object isn’t null (otherwise, the method will return null).
30.What is state management?
- This is most important question in flutter interview questions
- State Management: State Management is also called UI state or local state in Flutter data, and in Flutter it pertains to a particular widget. In another words, it is a state that update any value on time. By means of StatefulWidget, Flutter provides support for this state.
- App State: It is the state that we intend to share across different parts of the app and which we want to maintain between sessions.
31. What is the difference between Packages and Plugins?
- A Package contains only Dart code, while a Plugin contains both Dart & Native code, and it’s used to access native features. Usually on pub.dev, both packages and plugins are referred to as packages and only while creating a new package is the distinction clearly mentioned.
32.When is it appropriate to use packages, plugins, or third-party dependencies?
- In Flutter the Packages and plugins are best for use and saving you time and work. There’s no necessity to tackle a complex issue and Problem yourself when someone has already done it, especially if the solution is highly rated.
- Conversely, there’s a risk of over-reliance on third-party packages. They can become faulty, buggy, or even neglected over time. When you need to switch to a new package down the road, you might have to make huge changes to your code.
This is Top flutter interview questions