Flutter Beginner Tutorial For Mobile App Developers
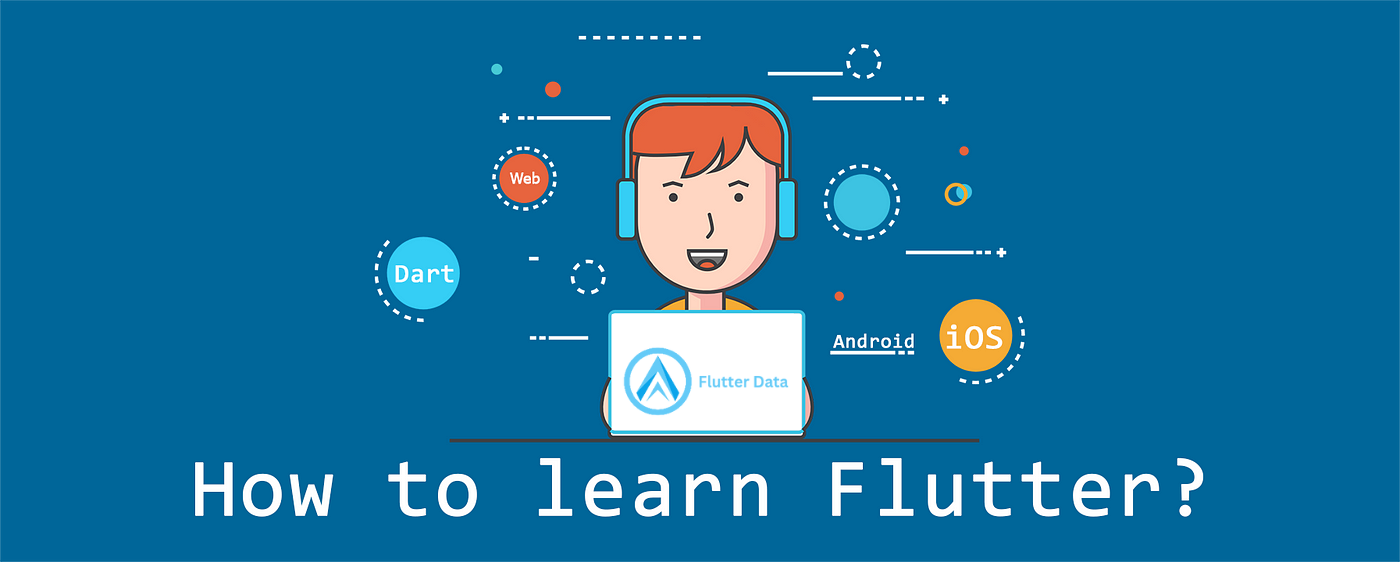
This Flutter Beginner Tutorial is your gateway to crafting beautiful and functional applications for both iOS and Android and other platform. With no prior programming experience required, you’ll gain a solid foundation in Flutter data, Google’s innovative framework designed for seamless cross-platform development.
By the end of this Flutter Beginner Tutorial, you’ll be equipped with the confidence and knowledge to embark on building real-world mobile applications. So, dive in and unlock the exciting possibilities of Flutter development!
Flutter Beginner Tutorial: Dive into Mobile App Development
What is Flutter?
Imagine a framework that allows you to develop high-performance mobile apps for both Android and iOS using a single codebase. That’s the magic of Flutter! Developed by Google, Flutter utilizes the Dart programming language to create visually appealing and feature-rich applications. (Flutter interview questions)
Why Choose Flutter for Mobile App Development?
There are compelling reasons to consider Flutter for your mobile app development journey:
- Cross-Platform Development:Flutter eliminates the need to write separate codebases for Android and iOS. With a single codebase, you can reach a wider audience across both operating systems, saving time and resources.
- Rich User Interfaces with Hot Reload: Flutter excels at crafting beautiful and intuitive user interfaces (UIs). The framework’s hot reload feature allows you to see changes in your code reflected in the app almost instantly, accelerating the development process significantly.
- Large and Active Community: The Flutter community is vibrant and ever-growing, offering a wealth of resources, tutorials, and forums for support. Whether you encounter a coding challenge or simply seek inspiration, the Flutter community is there to help you navigate your development journey.
Setting Up Your Flutter Development Environment
Before diving into the world of Flutter widgets and layouts, let’s ensure you have the necessary tools at your disposal.
Prerequisites
- A Computer: You’ll need a computer running Windows, macOS, or Linux to embark on your Flutter development adventure.
- A Text Editor or IDE: While a simple text editor can work, using an Integrated Development Environment (IDE) offers a more streamlined experience. Popular options include Android Studio (officially supported by Google) or Visual Studio Code.
Installing Flutter SDK
Head over to the official Flutter website and follow the detailed instructions for installing the Flutter SDK on your chosen operating system. The installation process is straightforward and shouldn’t take too long. (You Read the Flutter Beginner Tutorial)
Setting Up Your IDE Android Studio or VS Code
Once you have the Flutter SDK installed, configure your chosen IDE to recognize Flutter projects. Both Android Studio and VS Code offer plugins specifically designed for Flutter development.
Understanding the Building Blocks of Flutter Apps: Widgets
Now that your development environment is set up, let’s delve into the heart of Flutter applications: widgets.
Understanding the Building Blocks of Flutter Apps: WidgetsÂ
What are Widgets?
In Flutter, everything you see on the screen, from buttons and text to layouts and images, is built using widgets. Think of widgets as Lego bricks for your mobile app. They are the fundamental building blocks that you can combine and arrange to create complex and interactive user interfaces.
Each widget has a specific purpose and defines a part of your app’s UI. For instance, a Text widget displays text on the screen, while a Button widget allows users to interact with the app by triggering a specific action.
Types of Widgets
Flutter offers a rich library of pre-built widgets that cater to various functionalities. However, you can also create custom widgets to address specific needs within your app.
Here’s a breakdown of the two main categories of widgets in Flutter:
-
Stateful Widgets vs. Stateless Widgets:
- Stateful Widgets: These widgets hold internal state that can change over time. Imagine a toggle switch; its state can be either on or off. Stateful widgets are ideal for situations where the UI needs to update dynamically based on user interaction or changes in data.
- Stateless Widgets: These widgets represent a fixed state and cannot change after they are built. A simple text label displaying a greeting message is an example of a stateless widget.
Building Your First Widget: Text Widget
Let’s get your hands dirty by creating your first widget: a Text widget that displays a hello message!
- Open your IDE (Any Editor) and create a new Flutter project to build mobile app.
- Navigate to the lib/main.dart file, which is the main entry point of your app.
- Replace (Change code you already write) the existing code with these code are blow:
Dart
import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('My First Flutter App'), ), body: Center( child: Text('Hello World!'), ), ), ); } }
This code defines a MyApp class that extends StatelessWidget. The build method is responsible for returning the widget tree that constitutes your app’s UI.
In this example, we’ve nested several widgets:
- MaterialApp: This is the root widget for most Flutter apps and provides overall app-level functionalities.
- Scaffold: This widget serves as the basic layout for your app screen, including an app bar and a body section.
- AppBar: This widget creates the application bar at the top of the screen, displaying the app title in our case (My First Flutter App).
- Center: This widget, as the name suggests, centers its child widget within the available space.
- Text: Finally, the Text widget displays the much-anticipated “Hello World!” message on the screen.
- Save the changes and run the app on your preferred device or emulator. You should see a screen with the app bar displaying “My First Flutter App” and the centered text “Hello World!” congratulating you on your first steps into the world of Flutter development!
Congratulations! You’ve successfully created your first Flutter widget and witnessed the power of this framework in building user interfaces.
Crafting Layouts in Flutter
Now that you’ve grasped the concept of widgets, let’s explore how to arrange them to create visually appealing and well-structured layouts for your Flutter apps.
Understanding Layouts
Layouts define the overall organization of your app’s UI elements. They determine how widgets are positioned and sized on the screen, ensuring a cohesive and user-friendly experience.
Flutter provides a collection of built-in layout widgets that help you arrange your UI components effectively. These layout widgets act as containers for other widgets, defining their placement and how they interact with each other.
Common Layouts in Flutter
Here’s a glimpse into some of the most commonly used layout widgets in Flutter:
-
Row and Column Layouts:
- Row: This layout arranges its child widgets horizontally in a row, one after another, from left to right. Imagine a row of buttons or a list of items displayed horizontally.
- Column: This layout stacks its child widgets vertically, one on top of the other. A typical use case would be displaying labels and text fields stacked vertically within a form.
-
Stack Layout:
The Stack layout allows you to overlap multiple child widgets on top of each other. This is useful for creating layered effects or implementing functionalities like carousels where one element slides over another.
-
Padding and Margin:
While layout widgets define the overall arrangement, padding and margin offer finer control over the spacing between widgets. Padding adds space around the content of a widget, while margin adds space around the entire widget itself. These properties are crucial for creating a sense of balance and hierarchy in your app’s UI. Read more Flutter Beginner Tutorial
Experimenting with Layouts
The best way to solidify your understanding of layouts is to experiment! Try modifying the main.dart file from your previous example:
- Replace the Center widget with a Row widget:
Dart
body: Row( mainAxisAlignment: MainAxisAlignment.center, children: [ Text('Hello'), SizedBox(width: 20), // Add spacing between texts Text('World!'), ], ),
This code creates a horizontal row with the “Hello” and “World!” texts positioned side-by-side, centered within the available space using mainAxisAlignment: MainAxisAlignment.center.
- Play around with different layout widgets like Column and Stack to create various arrangements for your UI elements.
Remember, mastering layouts is essential for building user interfaces that are not only functional but also visually pleasing and intuitive.
In the next section, we’ll delve into adding interactivity to your Flutter apps with gestures!
Adding Interactivity with Gestures
A core aspect of any mobile app is interactivity. Users expect to be able to interact with the UI elements to navigate, trigger actions, and provide input. Flutter empowers you to incorporate this interactivity seamlessly through gestures.
Handling User Interaction: The Gesture Detector
The GestureDetector widget serves as the bridge between user gestures (taps, swipes, etc.) and your app’s functionality. It recognizes these gestures and allows you to define custom actions to be performed in response.
Here’s a basic example demonstrating a GestureDetector:
Dart
GestureDetector( onTap: () { print('You tapped the Text'); }, child: Text('Tap me!'), ),
In this example, the GestureDetector wraps a Text widget displaying “Tap me!”. When the user taps on the text, the onTap callback is triggered, printing a message to the console indicating the user interaction.
Implementing Common Gestures: Taps, Swipes, and More
Flutter supports a variety of gestures that you can leverage to create a rich and interactive user experience. Here are some commonly used gestures:
- Tap: This is the most basic gesture, signifying a single touch on the screen. You can utilize the onTap callback to handle tap events.
- Double Tap: As the name suggests, this gesture involves tapping the screen twice in quick succession. The onDoubleTap callback is used to define actions for this specific gesture.
- Swipe: Swiping gestures involve moving the finger across the screen in a specific direction (up, down, left, or right). You can implement callbacks like onSwipeUp, onSwipeDown, etc., to handle these directional swipes.
- Drag: Dragging involves holding a touch down and moving the finger across the screen. The onPanStart, onPanUpdate, and onPanEnd callbacks provide control over different stages of the drag gesture.
By effectively utilizing these gestures and their corresponding callbacks, you can create dynamic and engaging interactions within your Flutter apps.
Beyond the Basics: Advanced Gesture Handling
While the examples above showcase basic gesture recognition, Flutter offers more advanced functionalities. You can explore features like:
- Drag and Drop: Implement functionalities where users can drag UI elements and drop them onto designated targets.
- Multi-Touch Gestures: Handle situations where users interact with the screen using multiple fingers simultaneously (e.g., pinch to zoom).
- Gesture Recognition with Custom Logic: Define custom logic within callbacks to perform specific actions based on the gesture’s properties like speed, direction, or duration of the touch.
Exploring these advanced functionalities allows you to create intuitive and sophisticated user interactions within your Flutter apps.
In the next section, we’ll explore the concept of app state and how to manage it effectively in Flutter.
Managing App State in Flutter
Imagine an app that displays a counter that increments with each tap. This requires the app to remember the current count value, which is a dynamic piece of information that can change throughout the user’s interaction. This dynamic data is referred to as the app’s state.
Understanding App State
App state encompasses all the data that can change within your Flutter application. It includes values like user preferences, form input, or the current selection within a list. Effectively managing app state is crucial for building dynamic and responsive user interfaces.
Using StatefulWidgets for Dynamic UIs
While StatelessWidgets are excellent for static UI elements, they cannot hold or modify state. For scenarios where the UI needs to update based on changes in data, we leverage StatefulWidgets.
StatefulWidgets possess an internal state that can be modified over time. This state is typically represented by a Dart class that holds the relevant data properties.
The setState() Function
The setState function is the heart of managing state in StatefulWidgets. Whenever you modify the state of a StatefulWidget, you must call setState to notify the framework that the state has changed. This triggers a rebuild of the widget tree, ensuring the UI reflects the updated state values. Read more Flutter Beginner Tutorial
Here’s a simplified example demonstrating state management with a counter app:
Dart
class CounterApp extends StatefulWidget { @override _CounterAppState createState() => _CounterAppState(); } class _CounterAppState extends State<CounterApp> { int _counter = 0; void _incrementCounter() { setState(() { _counter++; }); } @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('Counter App'), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Text( 'Count: $_counter', style: TextStyle(fontSize: 24), ), ElevatedButton( onPressed: _incrementCounter, child: Text('Increment'), ), ], ), ), ), ); } }
In this example:
- The CounterApp class is a StatefulWidget that holds the current count value (_counter) as its state.
- The _incrementCounter method increases the counter value and then calls setState to trigger a UI rebuild.
- The build method displays the current count and an “Increment” button. Clicking the button calls _incrementCounter, updating the state and reflecting the change in the UI.
This is a very basic example, but it highlights the core principles of state management with StatefulWidgets and the setState function.
In the following section, we’ll delve into building your first complete Flutter app: “Hello World!” I shall guide you through creating a new Flutter project for Android and Ios and others Platform, understanding its coding structure, modifying the app UI, and finally, running your flutter app on a device or emulator.
Building Your First Flutter App: “Hello World!”
Now that you’ve grasped the fundamental concepts of widgets, layouts, gestures, and state management, it’s time to create your very first Flutter application! We’ll revisit the classic “Hello World!” program but with a Flutter twist.
Creating a New Flutter Project
- Open your preferred IDE (Android Studio or VS Code with the Flutter plugin installed).
- Navigate to the menu option for creating a new Flutter project (e.g., “File” -> “New” -> “Project” in Android Studio).
- Choose a project name (e.g., hello_world) and a project location on your computer.
- Click “Finish” to create the new Flutter project.
Understanding the Project Structure
Once your project is created, take a moment to familiarize yourself with the basic Flutter project structure. The lib directory contains the core Dart code for your app. The main.dart file is the entry point for your application. Read more Flutter Beginner Tutorial
Modifying the Main Content (Text Widget)
We’ll revisit the main.dart file and modify it to display our “Hello World!” message:
- Open the lib/main.dart file in your IDE.
- Replace (Change code you already write) the existing code with these code are blow:
Dart
import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('Hello World!'), ), body: Center( child: Text( 'Welcome to Flutter Development!', style: TextStyle(fontSize: 24), ), ), ), ); } }
In this code:
- We’ve replaced the initial “Hello World!” text with a more welcoming message: “Welcome to Flutter Development!”
- We’ve adjusted the font size to enhance readability.
Running the Flutter App on an Emulator or Real Mobile Device
- Connect your Android device or launch an emulator within your IDE.
- Click the “Run” button in your IDE to initiate the app deployment process.
- Select your target device or emulator from the available options.
- You should witness the app launch on your chosen device or emulator, displaying the “Hello World!” message and the app bar title.
Congratulations! You’ve successfully built, deployed, and run your first Flutter application. This might seem like a simple step, but it marks the beginning of your exciting journey into the world of Flutter app development.
Taking it Further with Flutter: Essential Resources
As you embark on your Flutter development path, here are some valuable resources to empower your learning:
- Official Flutter Documentation: The official Flutter documentation (https://docs.flutter.dev) is a comprehensive resource encompassing tutorials, code samples, and detailed explanations of Flutter concepts.
- Online Courses and Tutorials: Numerous online platforms offer high-quality Flutter courses and tutorials. Explore platforms like Udemy, Coursera, or Pluralsight to find courses that align with your learning style and goals.
- Flutter Community Resources: The Flutter community is vibrant and supportive. Engage in online forums like Stack Overflow or the official Flutter Community forum ([invalid URL removed]) to ask questions, share your progress, and learn from other developers.
By leveraging these resources and continuing to practice, you’ll progressively build your Flutter development skills and create more complex and feature-rich mobile applications.
Conclusion
This comprehensive Flutter beginner tutorial has equipped you with the foundational knowledge to begin your mobile app development journey with Flutter. You’ve explored core concepts like widgets, layouts, gestures, and state management. You’ve also successfully built your first Flutter app, witnessing the power and potential of this framework. Remember, consistent practice and exploration are key to mastering Flutter. As you delve deeper into its functionalities and explore advanced topics, you’ll unlock the ability to create stunning and engaging mobile experiences for users worldwide.
FAQs
-
What are the benefits of using Flutter for mobile app development?
Flutter offers several advantages, including:
- Cross-platform development for Android and iOS with a single codebase.
- Rich and visually appealing UIs with the ability to create custom widgets.
- Hot reload for rapid development cycles, allowing you to see changes reflected almost instantly.
-
What are some additional resources for learning Flutter?
In addition to the resources mentioned earlier, consider exploring YouTube channels dedicated to Flutter development. Many channels offer video tutorials and project